To create a blog in PHP and MySQL, set up a local server and create a database. Develop PHP scripts to handle CRUD operations.
Creating a blog using PHP and MySQL is a straightforward yet rewarding process for web developers. Start by installing a local server environment like XAMPP or WAMP, which includes Apache, PHP, and MySQL. This setup allows you to run PHP scripts and manage databases locally.
After setting up your server, create a MySQL database to store blog posts, user information, and other relevant data. Utilize PHP to write scripts that handle creating, reading, updating, and deleting (CRUD) blog posts. With these steps, you can build a functional blog from scratch, enhancing both your programming skills and online presence.
Setting Up Environment
Creating a blog using PHP and MySQL involves several steps. The first crucial step is setting up your development environment. This includes installing PHP and setting up MySQL. Proper setup ensures your blog runs smoothly and efficiently.
Installing Php
PHP is a server-side scripting language. To install it, follow these steps:
- Download the latest PHP version from the official PHP website.
- Follow the installation instructions based on your operating system.
- After installation, configure the PHP settings. Open the
php.ini
file and set appropriate values. - Verify the installation by running
php -v
in the command line.
Ensure you have the necessary extensions like mysqli enabled. These are vital for database interactions.
Setting Up Mysql
MySQL is a powerful database management system. Follow these steps to set it up:
- Download MySQL from the official MySQL website.
- Install MySQL by following the provided guidelines.
- After installation, open the MySQL Command Line Client.
- Create a new database for your blog using the command:
CREATE DATABASE blog_db;
- Create a user and grant permissions with the following commands:
CREATE USER 'blog_user'@'localhost' IDENTIFIED BY 'password';
GRANT ALL PRIVILEGES ON blog_db. TO 'blog_user'@'localhost';
FLUSH PRIVILEGES;
Now you have PHP and MySQL ready. Your development environment is set up for creating a blog.
Creating Database
Creating a database is a crucial step in setting up your blog in PHP and MySQL. Your database will store all the blog’s data, such as posts, comments, and user information. This section will guide you through creating the database, including setting up the tables needed for your blog.
Database Structure
The database structure is the backbone of your blog. It defines how data is stored and retrieved. A well-structured database ensures your blog runs efficiently.
Here’s a simple structure for your blog database:
- Posts Table: Stores blog posts with columns for ID, title, content, author, and date.
- Comments Table: Stores comments with columns for ID, post_id, author, content, and date.
- Users Table: Stores user information with columns for ID, username, password, email, and role.
Setting Up Tables
To set up tables in MySQL, you need to write SQL queries. Below are the SQL queries for creating the necessary tables for your blog.
Posts Table:
CREATE TABLE posts (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
content TEXT NOT NULL,
author VARCHAR(100) NOT NULL,
date TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Comments Table:
CREATE TABLE comments (
id INT AUTO_INCREMENT PRIMARY KEY,
post_id INT NOT NULL,
author VARCHAR(100) NOT NULL,
content TEXT NOT NULL,
date TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (post_id) REFERENCES posts(id)
);
Users Table:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
password VARCHAR(255) NOT NULL,
email VARCHAR(100) NOT NULL,
role VARCHAR(50) NOT NULL
);
Run these SQL queries in your MySQL database to create the tables. This setup will help manage your blog content efficiently.
Building The Frontend
Creating the frontend of your blog is exciting. It’s where design meets functionality. You’ll use HTML and CSS to make your blog look great and work well.
Html And Css Basics
HTML is the backbone of any webpage. It structures your content. Use HTML to create headers, paragraphs, lists, and more.
- Headers: Use to tags for titles.
- Paragraphs: Use the tag for text blocks.
- Lists: Use for unordered lists and for ordered lists.
CSS makes your blog beautiful. It styles the HTML elements. Use CSS to change colors, fonts, spacing, and layout.
- Selectors: Target elements using class, id, or type selectors.
- Properties: Use properties like
color
,font-size
, andmargin
. - Values: Assign values to properties, like
red
,16px
, or10px
.
Creating Blog Templates
Templates help you reuse code. They make your blog consistent. Create templates for your blog’s header, footer, and main content area.
Template Part | Description |
---|---|
Header | Includes your blog’s title and navigation links. |
Footer | Contains copyright information and additional links. |
Main Content | Displays your blog posts and articles. |
Use HTML for the structure of these parts. Then style them with CSS. Here’s a simple example of a header template:
My Blog
Style it with CSS for a polished look:
header {
background-color: #f8f8f8;
padding: 20px;
text-align: center;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin: 0 10px;
}
nav ul li a {
text-decoration: none;
color: #333;
}
With templates, your blog’s frontend will be neat and easy to manage.

Connecting To Database
Connecting to a database is crucial for dynamic blog content. PHP and MySQL make this easy. Let’s dive into the steps required to establish this connection.
Database Configuration
First, configure your database settings. This includes the database host, username, password, and database name. Use a configuration file to store these details securely.
Configuration Key | Example Value |
---|---|
Host | localhost |
Username | root |
Password | password123 |
Database Name | my_blog |
Establishing Connection
Now, let’s establish a connection. Use PHP’s mysqli_connect()
function. This function requires the configuration settings.
<?php
$host = 'localhost';
$username = 'root';
$password = 'password123';
$dbname = 'my_blog';
$conn = mysqli_connect($host, $username, $password, $dbname);
if (!$conn) {
die(‘Connection failed: ‘ . mysqli_connect_error());
}
echo ‘Connected successfully’;
?>
This code connects to the database. If it fails, it shows an error message. If successful, it shows “Connected successfully”.
Ensure to test this connection before proceeding. A successful connection means you can now interact with the database.
Developing Backend
Developing the backend for a blog in PHP and MySQL is crucial. It ensures your blog works smoothly and efficiently. The backend handles data storage, retrieval, and the logic behind your blog. In this section, we will focus on writing PHP scripts and handling form submissions.
Writing Php Scripts
PHP scripts form the backbone of your blog. They allow you to interact with the MySQL database.
First, create a connection to the MySQL database using PHP.
php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "blog_db";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn-connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
The above code connects your PHP script to the MySQL database. Replace blog_db with your database name.
Next, create PHP scripts to fetch and display blog posts.
php
$sql = "SELECT id, title, content FROM posts";
$result = $conn-query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "id: " . $row["id"]. " - Title: " . $row["title"]. " " . $row["content"]. "
";
}
} else {
echo "0 results";
}
$conn->close();
?>
Handling Form Submissions
Handling form submissions is key for adding new blog posts. Users need a way to submit data.
First, create an HTML form for submitting new posts.
The form sends data to submit_post.php. Now, write the PHP script to handle the form data.
php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "blog_db";
$title = $_POST['title'];
$content = $_POST['content'];
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn-connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "INSERT INTO posts (title, content) VALUES ('$title', '$content')";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "
" . $conn->error;
}
$conn->close();
?>
The above script inserts new blog posts into the database. It retrieves data from the form and uses SQL to insert it.
By writing PHP scripts and handling form submissions, you can create a dynamic blog. This ensures your blog is functional and user-friendly.
Implementing Crud Operations
To create a dynamic blog, you need CRUD operations. CRUD stands for Create, Read, Update, and Delete. These operations allow you to manage your blog posts. Let’s explore how to implement CRUD operations using PHP and MySQL.
Creating Posts
To create a new post, you need a form. This form will collect the post’s title, content, and author. Here is an example form:
In create_post.php, you will handle the form data:
<?php
$title = $_POST['title'];
$content = $_POST['content'];
$author = $_POST['author'];
$conn = new mysqli('localhost', 'username', 'password', 'database');
$sql = "INSERT INTO posts (title, content, author) VALUES ('$title', '$content', '$author')";
$conn->query($sql);
$conn->close();
?>
Reading Posts
To display posts, you need to fetch data from the database. Use the following code to retrieve and display posts:
<?php
$conn = new mysqli('localhost', 'username', 'password', 'database');
$sql = "SELECT FROM posts";
$result = $conn->query($sql);
while ($row = $result->fetch_assoc()) {
echo "
” . $row[‘title’] . “
“;
echo “
” . $row[‘content’] . “
“;
echo “
Author: ” . $row[‘author’] . “
“;
}
$conn->close();
?>
Updating Posts
To update a post, first, display the current data in a form. Here is an example:
Title:
Content:
In update_post.php, handle the form data and update the database:
<?php
$id = $_POST['id'];
$title = $_POST['title'];
$content = $_POST['content'];
$author = $_POST['author'];
$conn = new mysqli('localhost', 'username', 'password', 'database');
$sql = "UPDATE posts SET title='$title', content='$content', author='$author' WHERE id=$id";
$conn->query($sql);
$conn->close();
?>
Deleting Posts
To delete a post, you need a delete button. Here is an example:
In delete_post.php, handle the form data and delete the post:
<?php
$id = $_POST['id'];
$conn = new mysqli('localhost', 'username', 'password', 'database');
$sql = "DELETE FROM posts WHERE id=$id";
$conn->query($sql);
$conn->close();
?>
Adding Extra Features
Adding extra features to your blog enhances user experience. It also improves functionality and boosts engagement. Here are some key features to consider:
User Authentication
User authentication is essential for a secure blog. It allows users to create accounts and log in. Here’s a simple PHP code snippet to get you started:
php
session_start();
include('db.php');
if (isset($_POST['login'])) {
$username = $_POST['username'];
$password = $_POST['password'];
$query = "SELECT FROM users WHERE username='$username' AND password='$password'";
$result = mysqli_query($conn, $query);
if (mysqli_num_rows($result) == 1) {
$_SESSION['username'] = $username;
header("Location: dashboard.php");
} else {
echo "Invalid username or password";
}
}
?
Comment System
A comment system lets users interact with your content. It increases engagement. Below is a basic structure for adding comments:
php
if (isset($_POST['submit_comment'])) {
$comment = $_POST['comment'];
$post_id = $_POST['post_id'];
$username = $_SESSION['username'];
$query = "INSERT INTO comments (post_id, username, comment) VALUES ('$post_id', '$username', '$comment')";
mysqli_query($conn, $query);
}
?
Seo Optimization
SEO optimization helps your blog rank higher in search results. Here are some tips:
- Meta Tags: Add meta tags for keywords and descriptions.
- Headings: Use H1, H2, and H3 tags properly.
- Alt Text: Add alt text to images.
- Permalinks: Use SEO-friendly URLs.
Example of adding meta tags in PHP:
php
function add_meta_tags($title, $description) {
echo "<title$title";
echo "";
}
?>
php add_meta_tags('My Blog Post', 'This is a description of my blog post.'); ?
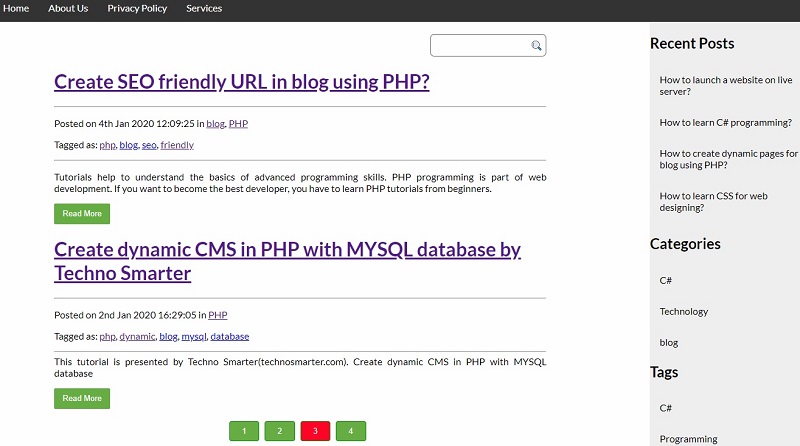
Credit: github.com
Frequently Asked Questions
How To Create Blogs In Php?
To create blogs in PHP, set up a PHP environment. Use a database like MySQL to store blog data. Create PHP scripts for adding, editing, and displaying blog posts. Design a user-friendly interface using HTML/CSS. Secure your application to prevent SQL injection and other vulnerabilities.
How To Create A Database Website With Php And Mysql?
Install PHP and MySQL. Create a MySQL database. Write PHP code to connect to the database. Design HTML forms to interact with the database. Test and deploy your website.
How To Create A Dynamic Web Page Using Php And Mysql?
To create a dynamic web page using PHP and MySQL, connect to a MySQL database using PHP. Use SQL queries to retrieve data. Embed the PHP code within HTML to display the data dynamically. Use PDO or MySQLi for secure database interactions.
Always sanitize inputs to prevent SQL injection.
How To Create A Connection Between Php And Mysql?
To connect PHP and MySQL, use the `mysqli_connect()` function. Provide the hostname, username, password, and database name. Example: “`php $connection = mysqli_connect(“localhost”, “username”, “password”, “database”); “` Check the connection status using: “`php if (!$connection) { die(“Connection failed: “. Mysqli_connect_error()); } “`
How To Start A Blog With Php?
Start by setting up a local server using XAMPP or WAMP, and create a PHP file for your blog.
Conclusion
Creating a blog with PHP and MySQL is straightforward. With practice, you’ll master the basics quickly. Follow each step carefully. Ensure your database is secure and optimized. Regularly update your content and software. Enjoy sharing your thoughts and connecting with readers worldwide.
Happy blogging!
Hi would you mind sharing which blog platform you’re working with?
I’m going to start my own blog soon but I’m having a tough time
making a decision between BlogEngine/Wordpress/B2evolution and
Drupal. The reason I ask is because your design seems different then most blogs and I’m
looking for something unique. P.S My apologies for being off-topic but I had to ask!
You can use wordpress easy to handle and you can customize everything as you want for your blog site. We are helping peoples to grow their business. If you need any help text us on direct whatsapp from our website. Thanks.
It’s actually a great and helpful piece of information. I am glad that you simply shared
this helpful info with us. Please stay us up to date like this.
Thanks for sharing.
You are most welcome 🙂
I’m not that much of a internet reader to be honest but your sites really nice, keep it up!
I’ll go ahead and bookmark your site to come back later. Many thanks
Thanks for your valuable comment.
I have read so many articles or reviews on the topic of the blogger lovers but this article is genuinely a pleasant paragraph, keep
it up.
Thank you so much 🙂
Incredible points. Outstanding arguments. Keep up the good spirit.
Sure, Thanks for your valuable comment.
Hey there! I simply want to give you a big thumbs up
for tthe great info you have hre on this post. I will bbe returning to your blog for more soon.
Sure, Thank you 🙂
Howdy! I just want to offer you a huge thumbs up for your excellent
info you have got right here on this post.
I’ll be returning to your blog for more soon.
Thanks and you are most welcome.
Thank you for the good writeup. It in fact was a amusement
account it. Look advanced to more added agreeable from you!
However, how can we communicate?
Thanks for your valuable comment, you can contact me on whatsapp from the website.
hello!,I love your writing very so much! proportion we keep in touch more approximately your article on AOL?
I need a specialist in this house to resolve my problem.
May be that is you! Having a look ahead to peer you.
Thank you so much 🙂